CECS 277
LAB ASSIGNMENT 8
Assigned date: 5/1
Due date: 5/8
25 points
Programming patterns are good ideas (something bigger than code) that can be used over and over again in programming projects. The MVC pattern is a good way to think about writing all GUI applications: it separates the application into three main classes that interact with each other to coordinate the entire application.
- Model: This class is the bridge between the control and the view. The user activates a control, which calls a method in the model to tell it to change state; after it does so, it notifies the view that its state has changed (at which point the view calls other methods in the model that supply it with the information it displays). The model initiates no actions: it accepts commands from the controller and processes them. One can use the same model with many different view/controller classes: one might allow the user a much easier way to interact with the application.
- View: This class coordinates the appearance of the GUI. It decides where all the controls and displays go (labels, text fields, buttons, etc.) When notified by the model that the model has changed its state, the view redisplays itself by calling methods in the model that return all the information that the view must display. A view class is typically long and boring from a programming perspective, because it contains lots of specifics about how the GUI looks (things like fonts, sizes, foreground/background colors, and the placement of its components requires lots of details). Writing one involves understanding lots of classes from the standard Java library and inheritance.
- Controller: This class collects together all the controls in the GUI. Whenever the user activates a control, it calls a method in the Model to tell it to change its state appropriately. The listeners specified in the Controller are complicated (but small) chunks of Java code that detect an action by the user and call the method in the Model that takes care of that action. Writing controllers involves understanding lots of classes from the standard Java library and inheritance.
|
Design the caculator below by using two different techniques:
- [10 points] Without MVC pattern
- [15 points] MVC pattern
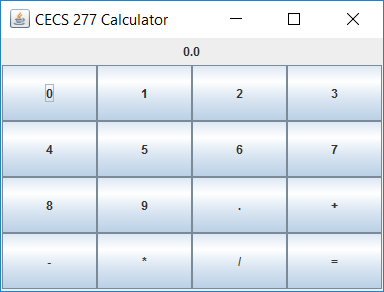
GRADING
- Be ready to demonstrate the result and answer some questions about the lab assignment.
- Submit the lab assignment to the BeachBoard.